本文最后更新于:December 5, 2021 pm
积土成山,风雨兴焉;积水成渊,蛟龙生焉;积善成德,而神明自得,圣心备焉。故不积跬步,无以至千里,不积小流无以成江海。齐骥一跃,不能十步,驽马十驾,功不在舍。面对悬崖峭壁,一百年也看不出一条裂缝来,但用斧凿,能进一寸进一寸,能进一尺进一尺,不断积累,飞跃必来,突破随之。
目录
1.for遍历
直接for进行遍历。不多说。╭(╯^╰)╮
2.直接赋值。
| int[] a={2,4,2,9,1,3,6,5,65,56,4,5,6,5}; int[] b = new int[200]; b = a; System.out.println(Arrays.toString(b));
|
3.数组的clone()方法
clone()方法我们在数组中是找不到的,它是object的方法,它的源码:
| protected native Object clone() throws CloneNotSupportedException;
|
使用方法:
| int[] a={2,4,2,9,1,3,6,5,65,56,4,5,6,5} int[] b= new int[50]; b = a.clone(); System.out.println(Arrays.toString(b));
|
4.System.arraycopy()方法(重点)
使用:public static native void arraycopy(Object src,int srcPos,Object dest,int desPos,int length)
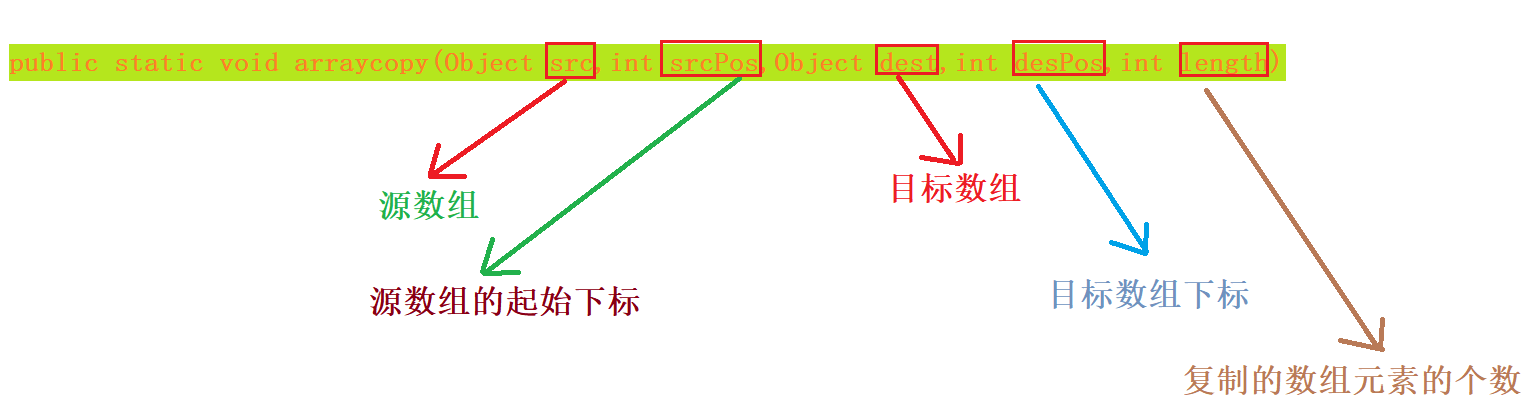
需要注意,新的数组多余的会自动补0。且System.arraycopy()比clone()方法快。
| int[] a={2,4,2,9,1,3,6,5,65,56,4,5,6,5}; int[] b = new int[20]; System.arraycopy(a,0,b,0,10); System.out.println(Arrays.toString(b)); System.arraycopy(a,0,b,0,a.length); System.out.println(Arrays.toString(a)); System.out.println(Arrays.toString(b)); System.out.println(b.length);
|
4.1 源码
| public static native void arraycopy(Object src, int srcPos, Object dest, int destPos, int length);
|
5.Arrays类的copyOf()方法和copyOfRange()方法
5.1 Arrays.copyOf()方法
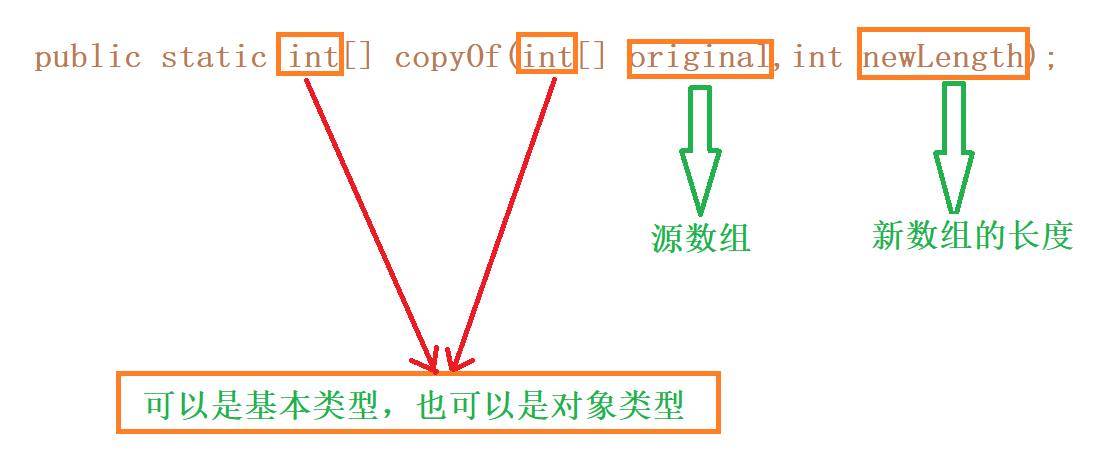
很显然,
如果newLength小于源数组的长度,则将源数组的前面若干个元素复制到目标数组。
如果newLength大于源数组的长度,则将源数组的所有元素复制到目标数组(这种方式的数组需要注意,见样例)。
它在方法内部调用了System.arraycopy(),只是相当于重写了一下,换了一个名字。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| int[] a={2,4,2,9,1,3,6,5}; int[] b = new int[303]; b = Arrays.copyOf(a,5); System.out.println(Arrays.toString(a)); System.out.println(Arrays.toString(b)); b = Arrays.copyOf(a,15); System.out.println(Arrays.toString(a)); System.out.println(Arrays.toString(b)); System.out.println(b.length);
|
5.1.1 源码
| public static int[] copyOf(int[] original, int newLength) { int[] copy = new int[newLength]; System.arraycopy(original, 0, copy, 0, Math.min(original.length, newLength)); return copy; }
|
5.2 copyOfRange()方法
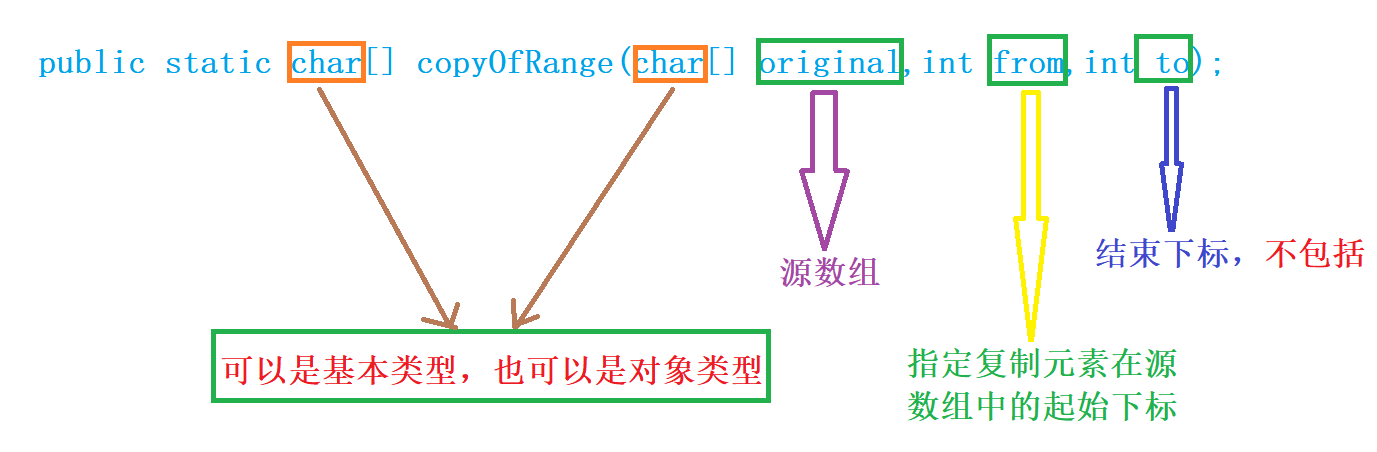
| int[] a={2,4,2,9,1,3,6,5,43,34,2,1}; int[] b = new int[303]; b = Arrays.copyOfRange(a,1,8); System.out.println(Arrays.toString(a)); System.out.println(Arrays.toString(b));
|
5.2.1 源码
| public static int[] copyOfRange(int[] original, int from, int to) { int newLength = to - from; if (newLength < 0) throw new IllegalArgumentException(from + " > " + to); int[] copy = new int[newLength]; System.arraycopy(original, from, copy, 0, Math.min(original.length - from, newLength)); return copy; }
|
可自行查看这些方法的源码。