本文最后更新于:May 13, 2023 pm
JSP标准标签库(JSTL)是一个JSP标签集合,它封装了JSP应用的通用核心功能。JSTL支持通用的、结构化的任务,比如迭代,条件判断,XML文档操作,国际化标签,SQL标签。 除了这些,还提供了一个框架来使用集成JSTL的自定义标签。
目录
需要进行导入 standard.jar 包和 jstl.jar 包。jakarta-taglibs-standard-1.1.2.zip 下载地址
1.JSTL标准标签库
可以对EL获取到的数据进行逻辑操作,与EL合作完成数据的展示。
1.1 JSTL的使用
在JSP页面引入标签库: <% @taglib uri=”http://java.sun.com/jsp/jstl/core" prefix=”c” >
注意:引入的是在jsp下的jstl !!!
2.JSTL核心标签
2.1 条件标签判断(if)
<c:if test=”条件”> < /c:if> 。test属性中是条件,但条件需要使用EL表达式来写。更重要的是,没有else 标签。
示例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| <%-- Created by IntelliJ IDEA. User: DragonOne Date: 2021/8/24 Time: 11:50 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <html> <head> <title>Title</title> </head> <body> <% request.setAttribute("num",123); %>
${num} <br> <c:if test="${num > 100}" > <h1>这是一个大于100的数:${num}</h1> </c:if>
</body> </html>
|
注意:在导包运行后可能存在报错问题,如下。
出错原因:在Artifacts中没有添加上jar包。
解决办法:
在Project Structure中进行设置,(第五步需要右击项目才会显示)如下。
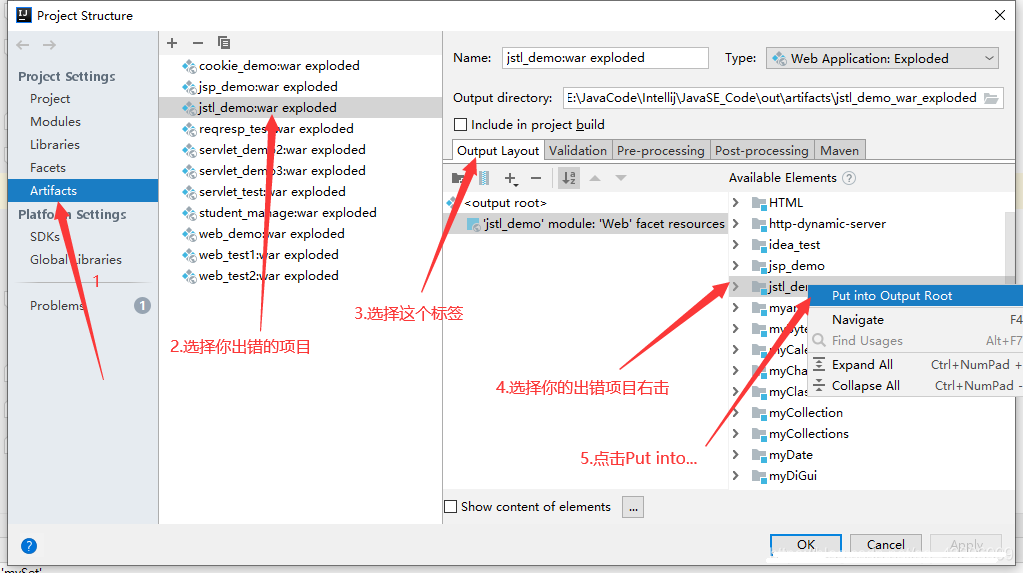
2.2 多条件判断(choose)
用法:
| <c:choose> <c:when test="条件1">结果1</c:when> <c:when test="条件2">结果2</c:when> <c:when test="条件3">结果3</c:when> <c:otherwise>结果4</c:otherwise> //当其他都不满足时执行 </c:choose>
|
示例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| <%-- Created by IntelliJ IDEA. User: DragonOne Date: 2021/8/24 Time: 11:50 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <html> <head> <title>Title</title> </head> <body> <% request.setAttribute("num",123); %>
${num} <br> <c:choose> <c:when test="${num < 100}"><h1>小于100</h1></c:when> <c:when test="${num > 200}"><h1>大于200</h1></c:when> <c:otherwise><h1>在100到200之间</h1></c:otherwise> </c:choose>
</body> </html>
|
2.3 迭代标签(foreach)
用法:
| <c:foreach var="变量名" items="集合名" begin="起始下标" end="结束下标" step="间隔长度" varstatus="遍历状态" > </c:foreach>
|
类似于:for(var it : items)
其中,varstatus 表示当前var对象的状态,主要有四种:
- first:是否是第一行。
- last:是否是最后一行。
- count:当前处于第几行。
- index:当前元素的下标。
示例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| <%@ page import="java.util.ArrayList" %> <%@ page import="java.util.*" %><%-- Created by IntelliJ IDEA. User: DragonOne Date: 2021/8/24 Time: 11:50 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <html> <head> <title>Title</title> </head> <body> <% request.setAttribute("num",123); List<String> list = new ArrayList<>(); list.add("A"); list.add("B"); list.add("C"); list.add("D"); request.setAttribute("list",list); %>
<c:forEach var="it" items="${list}" begin="0" end="3" step="1" varStatus="flag"> <h1>${it} ${flag.count} ${flag.index} ${flag.first} ${flag.last}</h1> </c:forEach>
</body> </html>
|
2.4 URL标签
示例:
| <c:url context="${pageContext.request.contextPath}" value="/xxx/abc.jsp" />
|
value 是连接在 context 后面的其他路径。